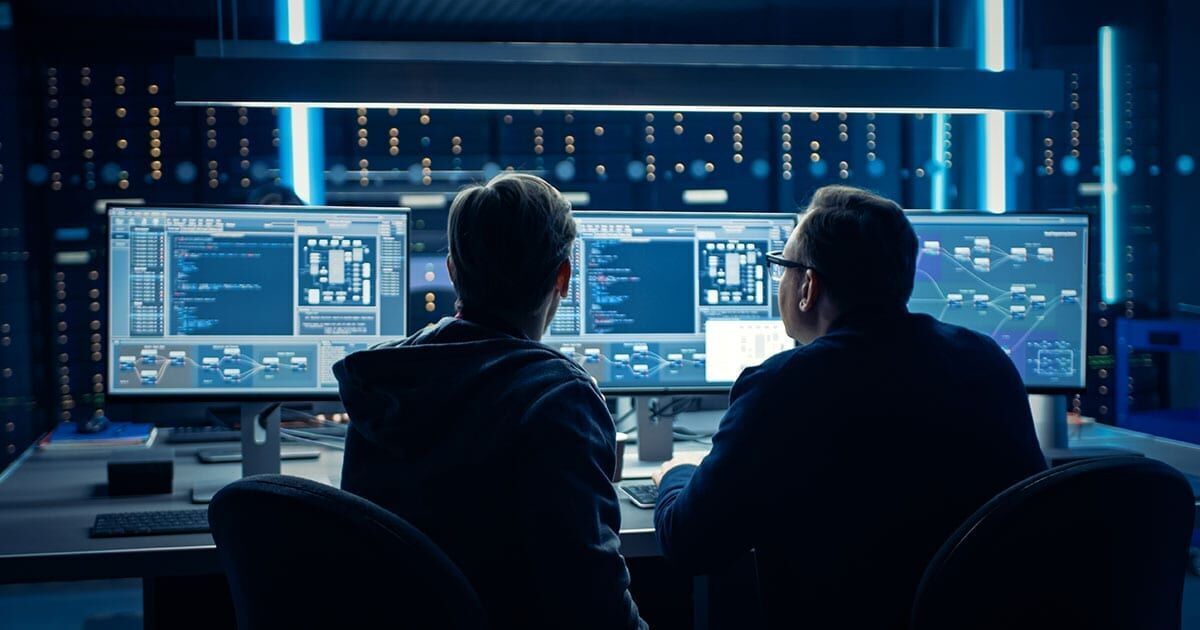
In web development, achieving seamless frontend experiences often relies on robust backend support. The Telerik library, which is an essential component that plays a vital role as a powerful ally for developers, seamlessly integrates with frontend stacks that support Kendo UI and provides streamlining data to enhance server-side operations for a wide array of UI components, including grids, charts, and many more. One drawback is that some services are free and the important packages require a commercial license, even though they offer a one-month free trial.
Key Features
Now, let’s dive into some of the key features that make Telerik Library an indispensable tool for backend development:
- Server-Side Data Operations: This library enables server-side data operations, which significantly reduces the burden of processing data on the client side. This leads to enhanced performance and a smoother user experience.
- Paging, Sorting, and Filtering: The Telerik library provides native support for essential features such as pagination, sorting, filtration, grouping, and many more. This means developers can implement these functionalities effortlessly, without the need for extensive custom coding on the client side.
- Enhanced Efficiency: Developers can unlock a new level of efficiency in backend development by leveraging the Telerik library, since this library’s comprehensive feature set minimizes the need for manual intervention. Thus, it allows programmers to focus on crafting innovative solutions rather than wrestling with backend intricacies.
- Seamless Integration: The Telerik library harmonizes effortlessly with Kendo UI, facilitating automatic data mapping without custom coding. Even in non-Kendo-supported frontend stacks, the library ensures smooth data operations. So, we can have custom mapping for seamless integration across diverse tech stacks.
Overview of Telerik Library
- Data Operations: It offers robust data management capabilities, including built-in data binding, pagination, sorting, filtration, and grouping functionalities for calculating aggregate values like sums, avg, min and max, etc., and many more.
- Data Validations: This framework typically offers excellent support for data validation and enforcing business rules for their entity models to meet specific criteria and constraints on the backend.
- Caching Mechanism: Its backend tools often incorporate caching techniques to optimize performance by storing frequently accessed data in memory, reducing the need for repeated database queries and improving overall application responsiveness.
- Data Access: It provides libraries and tools to streamline data access on the backend. This includes ORM (Object-Relational Mapping) frameworks like Telerik Data Access, which allows developers to work with databases using strongly-typed.NET objects.
- Data Entity Mapping: It provides tools for data modeling, mapping, and defining entity models that represent their data structure in code, and this simplifies CRUD (Create, Read, Update, Delete) operations by providing a clear abstraction layer.
- Security Mechanisms: It places a strong emphasis on security, and its backend tools typically include features for implementing authentication and authorization mechanisms.
Supporting Technologies
Frontend Technologies using Kendo
- AngularJs
- ReactJs
- VueJs
- .Net Razor
- .Net Blazor
- .Net MAUI
Backend Technologies using Telerik
- .Net Core MVC
- .Net Core WebApi
- Java (JSP, Servlets)
- PHP
- NodeJs
Comparison of Telerik with Other Libraries
Why choose Telerik
- Kendo UI optimization: The Telerik backend library specifically formats data for Kendo UI, ensuring a seamless and efficient integration.
- Ease of Use: The API is user-friendly, making it easier for developers to implement and manage data operations.
- Performance: We optimize the controls to ensure smooth and efficient operation of applications.
- Customization: High level of customization allows developers to tailor the controls to meet specific needs, enhancing the flexibility of the tool.
- Integration: Designed to work perfectly with Kendo UI, making it an ideal choice for projects using this UI library.
- Comprehensive documentation: A large community and detailed documentation provide significant support, making problem-solving easier.
- Competitive Pricing: Telerik offers a variety of licensing options that can fit different budgets, providing excellent value for the money.
Best Practices with Telerik
- Version Compatibility: Always ensure that the versions of Telerik libraries you’re using are compatible with the version of the backend project you’re working on. Telerik frequently releases updates and patches, so keeping your libraries up-to-date is crucial for stability, security, and the improvement of existing features.
- Proper Setup & Configuration: Follow Telerik’s official documentation and guidelines for setting up and configuring their libraries in your backend project. This includes adding necessary dependencies, configuring routes, and integrating client-side components with server-side code, etc.
- Optimised Data Handling: Utilize Telerik’s data components effectively to handle data operations efficiently. This includes using data grids, charts, and other components to display and manipulate data on the client side while ensuring smooth communication with the backend through APIs.
- Community Engagement & Support: Engage with the Telerik community, forums, and support channels to seek assistance, share knowledge, and stay updated on best practices and the latest developments related to Telerik libraries and your backend project.
Telerik Setup with .Net Core
We have many backend technologies that are supported by Telerik, but I will explain the Telerik setup with .Net Core. I’m attaching the official documentation link for Telerik Setup with.Net Core.
- Create a.Net Core project called
KendoWebApi
with either WebApi or MVC. - Under NuGet Package Manager, the only package source available is
nuget.org.
- Download the UI for the ASP.NET Core free trial installer. Then, create an account to access the installer file.
- When the installer is launched, it prompts users to select the desired products for installation based on their requirements. I have chosen
Telerik UI for ASP.NET Core
to meet the requirements of my project.
- After installing the product, go back to the project’s NuGet Package Manager. You can see
TelerikNuGetV3
added in Package Source. Install theTelerik.DataSource.Trail
to obtain native functionality for pagination, sorting, filtration, grouping, and additional features.
Implementation with Telerik
In this demonstration, we will explore the implementation of key built-in features of Telerik. This includes pagination, sorting, filtration, and grouping utilizing remote data sourced from a mock API.
Step 1: Open the KendoWebApi project and create Employee.cs
under Models with the necessary fields. Get the data from a mock API and use DataEnvelope<T>
to return the generic data.
using Telerik.DataSource;
namespace KendoWebApi.Models
{
public class Employee
{
public int Id { get; set; }
public string FullName { get; set; }
public string Email { get; set; }
public string Gender { get; set; }
public DateTime DateOfJoining { get; set; }
public int Experience { get; set; }
public string Country { get; set; }
}
public class DataEnvelope
{
public List GroupedData { get; set; }
public List CurrentPageData { get; set; }
public int TotalItemCount { get; set; }
public static implicit operator DataEnvelope(DataEnvelope v)
{
throw new NotImplementedException();
}
}
}
Step 2: Create a DataSourceService.cs
file to have our implementation requirements for the controller. Here, we will add the method GetResponseFromHttpRequest
to fetch data from the mock API using IHttpClientFactory.
.
using KendoWebApi.Models;
using Newtonsoft.Json;
namespace KendoWebApi.Services
{
public class DataSourceService : IDataSourceService
{
private readonly IHttpClientFactory _httpClient;
public DataSourceService(IHttpClientFactory httpClient)
{
_httpClient = httpClient;
}
public async Task<List> GetResponseFromHttpRequest()
{
string apiUrl = "https://mocki.io/v1/eaee4f4e-2d0a-48d3-87d3-1d99190474bd";
try
{
// Make a GET request
var httpResponse = await _httpClient.CreateClient().GetAsync(apiUrl);
// Check if the request was successful
httpResponse.EnsureSuccessStatusCode();
// Deserialise the response content into List of Employee
var apiResponse = await httpResponse.Content.ReadAsStringAsync();
var result = JsonConvert.DeserializeObject<List>(apiResponse);
return result;
}
catch (ApplicationException ex)
{
throw new ApplicationException($"Application Expection occurred - Message: {ex.Message}");
}
catch (Exception ex)
{
throw new Exception($"System Exception occurred - Message: {ex.Message}");
}
}
}
}
Step 3: Then, we will add a method to the DataSourceService.cs named GetResponseFromDataOperations,
leveraging the ToDataSourceResultAsync.
This orchestrates the execution of various data operations, transforming the mock data into a queryable format using AsQueryable.
If the requirement only involves data manipulation for pagination, sorting, and filtration
, we assign the data to CurrentPageData.
Alternatively, if grouping functionality is necessary
, the data should be cast to AggregateFunctionsGroup
and assigned to GroupedData.
public async Task<DataEnvelope> GetResponseFromDataOperations(DataSourceRequest dataSourceRequest, List employeeResponse)
{
try
{
DataEnvelope dataToReturn;
var processedData = await employeeResponse.AsQueryable().ToDataSourceResultAsync(dataSourceRequest);
// If grouping is necessary, then cast to AggregateFunctionsGroup
if (dataSourceRequest.Groups != null && dataSourceRequest.Groups.Any())
{
dataToReturn = new DataEnvelope
{
GroupedData = processedData.Data.Cast().ToList(),
TotalItemCount = processedData.Total
};
}
else
{
dataToReturn = new DataEnvelope
{
CurrentPageData = processedData.Data.Cast().ToList(),
TotalItemCount = processedData.Total
};
}
return dataToReturn;
}
catch (ApplicationException ex)
{
throw new ApplicationException($"Application Expection occurred - Message: {ex.Message}");
}
catch (Exception ex)
{
throw new Exception($"System Exception occurred - Message: {ex.Message}");
}
}
Step 4: Create anIDataSourceService.cs
file to provide an interface for the methods we use in DataSourceService.
using KendoWebApi.Models;
using Telerik.DataSource;
namespace KendoWebApi.Services
{
public interface IDataSourceService
{
Task<List> GetResponseFromHttpRequest();
Task<DataEnvelope> GetResponseFromDataOperations(DataSourceRequest dataSourceRequest, List employeesResponse);
}
}
Step 5: Then we need to add the lifetime scope for the DataSourceService and its interface to ‘Program.cs’.
builder.Services.AddScoped<IDataSourceService, DataSourceService>();
Step 6: Finally, we will create a KendoWebApiController.cs file to have our API call GetResponseFromHttpRequest
to fetch the data from the mock API, pass the data to ‘GetResponseFromDataOperations’, and return the data based on the data operations mentioned in the DataSourceRequest.
using KendoWebApi.Models;
using KendoWebApi.Services;
using Microsoft.AspNetCore.Mvc;
using Telerik.DataSource;
namespace KendoWebApi.Controllers
{
[Route("api/[controller]")]
[Produces("application/json")]
[ApiController]
public class KendoWebApiController : ControllerBase
{
private readonly IDataSourceService _dataSourceService;
public KendoWebApiController(IDataSourceService dataSourceService)
{
_dataSourceService = dataSourceService;
}
[HttpPost("GetFilteredEmployees")]
[Produces("application/json")]
public async Task<ActionResult<DataEnvelope>> GetFilteredEmployees([FromBody] DataSourceRequest dataSourceRequest)
{
try
{
var apiResponse = await _dataSourceService.GetResponseFromHttpRequest();
if (apiResponse == null || !apiResponse.Any())
{
return NotFound("No response found from this request");
}
var result = await _dataSourceService.GetResponseFromDataOperations(dataSourceRequest, apiResponse);
return Ok(result);
}
catch (ApplicationException exception)
{
throw new ApplicationException($"method execution failed with Business Exception - Message: {exception.Message}");
}
catch (AggregateException exception)
{
throw new AggregateException($"method execution failed with Aggregate Exception - Message: {exception.Message}");
}
catch (Exception exception)
{
throw new Exception($"method execution failed with System Exception - Message: {exception.Message}");
}
}
}
}
Conclusion
In conclusion, the Telerik library significantly enhances backend efficiency for web development by offering robust data operations, seamless integration with Kendo UI, and essential features such as pagination, sorting, filtering, and grouping. Its user-friendly API, optimized performance, and extensive documentation make it a valuable tool for developers.
Telerik provides a 30-day trial, giving developers the opportunity to gain knowledge and experience. While some advanced features require a commercial license after the trial period, the library’s comprehensive capabilities and support for various tech stacks justify the investment. Whether used with .NET Core or other backend technologies, Telerik streamlines development processes, allowing developers to focus on creating innovative solutions with minimal manual intervention.
Reference
Github Source: Codebase on GitHub for Telerik with .Net Core
Postman Collection’s JSON: DataSourceRequest Collection
Telerik Setup Demo: Demo Video to Setup Telerik with .Net Core