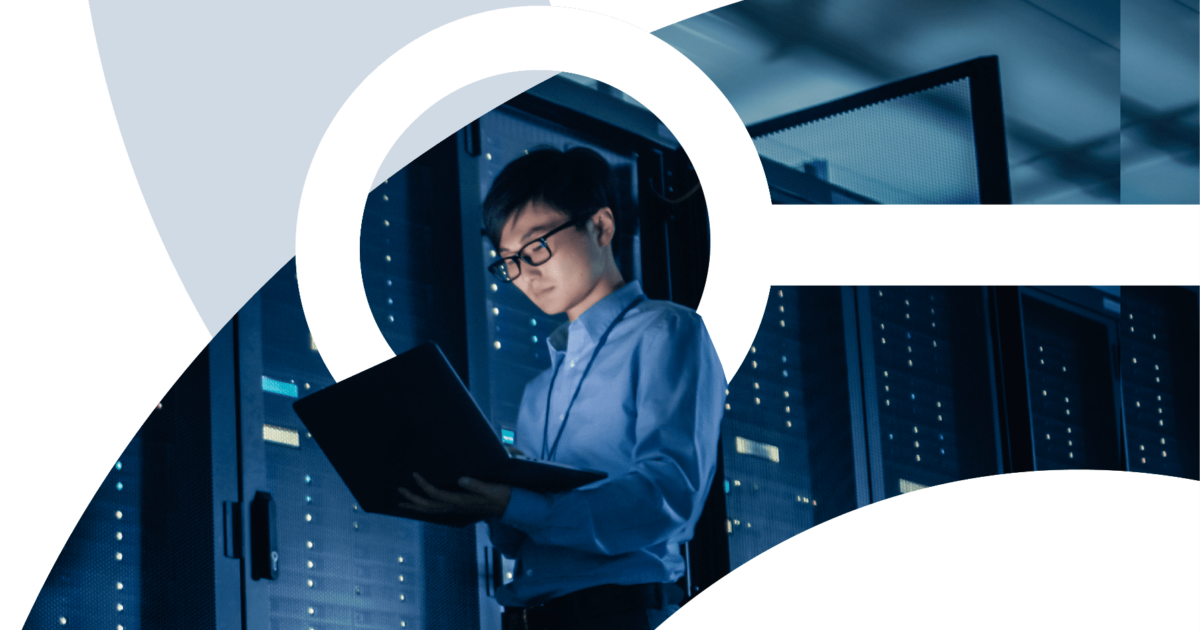
Consumer-driven Contract Testing is an approach where service consumers define the contract for their interactions with the service provider. This approach ensures that service providers meet the consumer’s expectations, as the contract defines the expected behavior of the provider’s service. In this blog, we will explore on how to perform consumer-driven contract testing using Postman, a popular API development and testing tool.
Let’s consider having a service provider that exposes an API to retrieve a list of books. The API has a GET endpoint with the following URL:
https://book-service-provider.com/books
The API returns a JSON array of book objects, with each book object containing the following attributes:
- Title (Title of the book)
-
Author (Author of the book)
- Published date (Publication date of the book)
- Publisher (Publisher of the book)
We will create a consumer contract test for this API using Postman.
Step 1: Define the Contract
The first step in consumer-driven contract testing is to define the contract. The contract should specify the expected behavior of the service provider’s API. In this example, we expect the API to return a JSON array of book objects, with each book object containing the attributes mentioned above.
Step 2: Write the Test Script
Next, we should write a test script in Postman to verify if the service provider’s API meets the contract.
We will then use Postman’s built-in testing framework to write the test script.
To start, we will create a new collection in Postman called “Book Service Consumer Contract Tests”. Within this collection, we will create a new request called “Get Books”.
Next, we will add a test script to the “Get Books” request to the API endpoint and verify if the response meets the contract.
// Verify the response schema
pm.test(“Response schema is valid”, function() {
const schema = {
“type”: “array”,
“items”: {
“type”: “object”,
“properties”: {
“title”: {“type”: “string”},
“author”: {“type”: “string”},
“published_date”: {“type”: “string”, “format”: “date-time”},
“publisher”: {“type”: “string”}
},
“required”: [“title”, “author”, “published_date”, “publisher”]
}
};
pm.response.to.have.jsonSchema(schema);
});
// Verify the response status code
pm.test(“Response status code is 200”, function() {
pm.response.to.have.status(200);
});
// Verify the response content type
pm.test(“Response content type is application/json”, function() {
pm.response.to.have.header(“Content-Type”, “application/json”);
});
The test script uses the jsonSchema assertion to verify that the response schema matches the contract we defined earlier. It also checks if the response is successful.
Step 3: Share the Contract with the Provider
Once we have defined the contract and written the test script, we need to share the contract with the service provider. We can export the contract from Postman in OpenAPI format and send it to the provider.
To export the contract, navigate to the “Get Books” request and click on the “Code” button. From there, we can select “Generate Code” and choose “OpenAPI” as the output format. This will generate an OpenAPI specification file that can be sent to the provider.
Conclusion
Consumer-driven contract testing is an important approach to ensure that service providers meet the expectations of their consumers. By defining the contract and writing the test script, consumers can verify that the provider’s API meets the contract.
Postman is a powerful tool for consumer-driven contract testing, providing built-in testing capabilities and the ability to export contracts in OpenAPI format. With Postman, consumers can easily define and test contracts, and share them with their service providers. By using this method, service providers can improve their API design and ensure that their consumers are satisfied with their services.